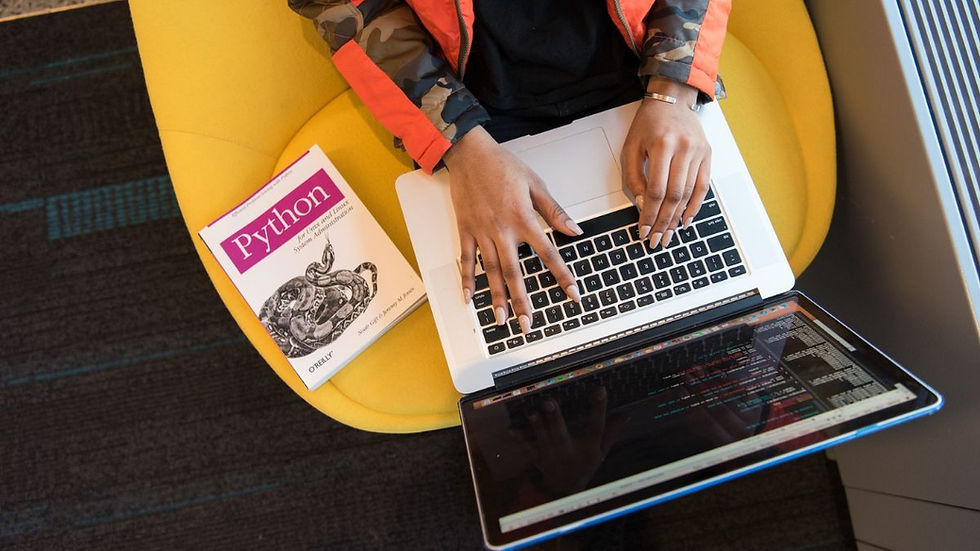
To make an HTTP request in Python, you can use the requests library. Here's an example of how to send a GET request to the Python documentation website:
import requests
response = requests.get('https://docs.python.org/3/')print(response.status_code) # Prints the status code of the responseprint(response.text) # Prints the contents of the response
You can also send other types of HTTP requests, such as POST, PUT, DELETE, etc. For example, here's how you would send a POST request:
import requests
data = {'key': 'value'}
response = requests.post('https://httpbin.org/post', data=data)print(response.status_code) # Prints the status code of the responseprint(response.text) # Prints the contents of the response
You can also include headers and other options in your request by passing them as arguments to the request function. For example:
import requests
headers = {'Content-Type': 'application/json'}
response = requests.post('https://httpbin.org/post', json={'key': 'value'}, headers=headers)print(response.status_code) # Prints the status code of the responseprint(response.text) # Prints the contents of the response
For more information, you can read the documentation for the requests library at https://requests.readthedocs.io/.
Comments