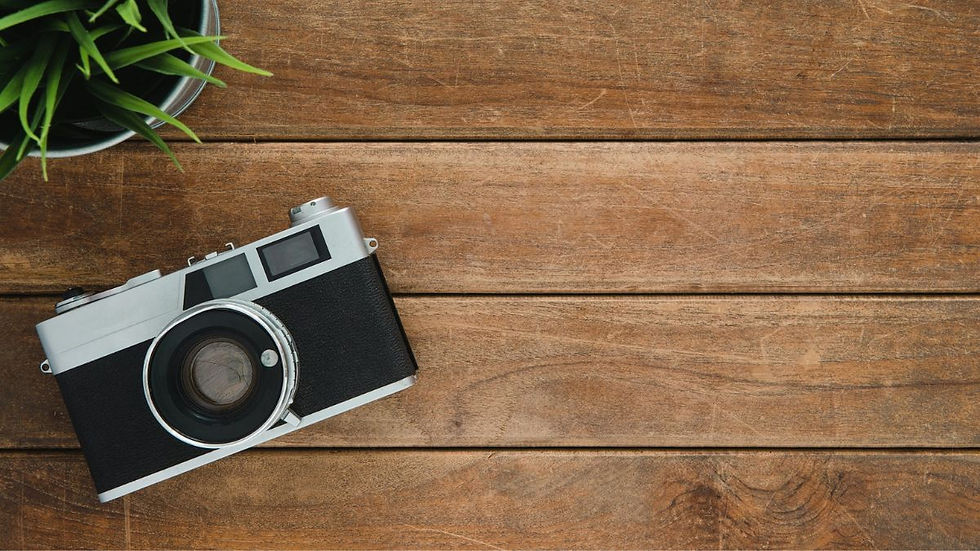
You can use the Python PIL (Python Imaging Library) module to resize an image. Here is an example of how to use PIL to resize an image and save the resized image to a new file:
from PIL import Image
# Open the image
im = Image.open('input.jpg')# Resize the image
im_resized = im.resize((200, 200))# Save the resized image to a new file
im_resized.save('output.jpg')
This example will open the image stored in the file input.jpg, resize it to a width and height of 200 pixels, and save the resized image to a new file called output.jpg.
You can also use the thumbnail() method to resize an image while preserving its aspect ratio. For example:
# Resize the image while preserving its aspect ratio
im.thumbnail((200, 200))# Save the resized image
im.save('output.jpg')
This will resize the image to a maximum width and height of 200 pixels, while preserving the aspect ratio of the original image. If the original aspect ratio is not the same as the target aspect ratio, the resulting image will be padded with transparent pixels to maintain the aspect ratio.
Comments